How To Declare A String In Dev C++
- C++ Declare String Variable
- How To Declare A String In Dev C Download
- Java Declare String Variable
- String In Dev C++
- How To Declare A String In Dev C File
- C++ Declare Array Of Strings
- Declare A String Java
- C++ Basics
As you can see in the example above, compound types are used in the same way as fundamental types: the same syntax is used to declare variables and to initialize them. For more details on standard C strings, see the string class reference. You are declaring a pointer that points to a string stored some where in your program (modifying this string is undefined behavior) according to the C programming language 2 ed. Char p2 = 'String'; You are declaring an array of char initialized with the string 'String' leaving to the compiler the job to count the size of the array.
- Jun 25, 2003 You will have to declare variables to input the data. Luckily, declaring variables in C is very easy. This simple program gets the user's age as input and displays it back to the screen. Here is the source code: #include using namespace std; int main int age; cout C Program'.
- THE WORLD'S LARGEST WEB DEVELOPER SITE. Declare Variables Declare Multiple Variables Identifiers Constants. C User Input C Data Types. Strings are used for storing text. A string variable contains a collection of characters surrounded by double quotes: Example.
- The last string of the group, useThisOneAgain, is initialized using an existing C string object. Put another way, this example illustrates that string objects let you do the following: Create an empty string and defer initializing it with character data. Initialize a string by passing a literal, quoted character array as an argument to the.
- The C-style character string. The string class type introduced with Standard C. The C-Style Character String. The C-style character string originated within the C language and continues to be supported within C. This string is actually a one-dimensional array of characters which is terminated by a null character ' 0'.
- THE WORLD'S LARGEST WEB DEVELOPER SITE. Strings Concatenation Numbers and Strings String Length Access Strings User Input Strings Omitting Namespace. To define a function outside the class definition, you have to declare it inside the class and then define it outside of the class.
- C++ Object Oriented
- C++ Advanced
- C++ Useful Resources
- Selected Reading
C++ provides following two types of string representations −
- The C-style character string.
- The string class type introduced with Standard C++.
The C-Style Character String
The C-style character string originated within the C language and continues to be supported within C++. This string is actually a one-dimensional array of characters which is terminated by a null character '0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word 'Hello'. To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word 'Hello.'
If you follow the rule of array initialization, then you can write the above statement as follows −
Following is the memory presentation of above defined string in C/C++ −
Actually, you do not place the null character at the end of a string constant. The C++ compiler automatically places the '0' at the end of the string when it initializes the array. Let us try to print above-mentioned string −
When the above code is compiled and executed, it produces the following result −
C++ supports a wide range of functions that manipulate null-terminated strings −
Sr.No | Function & Purpose |
---|---|
1 | strcpy(s1, s2); Copies string s2 into string s1. |
2 | strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | strlen(s1); Returns the length of string s1. |
4 | strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
Following example makes use of few of the above-mentioned functions −
When the above code is compiled and executed, it produces result something as follows −
The String Class in C++
The standard C++ library provides a string class type that supports all the operations mentioned above, additionally much more functionality. Let us check the following example −
When the above code is compiled and executed, it produces result something as follows −
What is a String?
String is nothing but a collection of characters in a linear sequence. 'C' always treats a string a single data even though it contains whitespaces. A single character is defined using single quote representation. A string is represented using double quote marks.
'C' provides standard library <string.h> that contains many functions which can be used to perform complicated string operations easily.
In this tutorial, you will learn-
Declare and initialize a String
A string is a simple array with char as a data type. 'C' language does not directly support string as a data type. Hence, to display a string in 'C', you need to make use of a character array.
The general syntax for declaring a variable as a string is as follows,
The classic string declaration can be done as follow:
The size of an array must be defined while declaring a string variable because it used to calculate how many characters are going to be stored inside the string variable. Some valid examples of string declaration are as follows,
The above example represents string variables with an array size of 15. This means that the given character array is capable of holding 15 characters at most. The indexing of array begins from 0 hence it will store characters from a 0-14 position. The C compiler automatically adds a NULL character '0' to the character array created.
Let's study the initialization of a string variable. Following example demonstrates the initialization of a string variable,
In string3, the NULL character must be added explicitly, and the characters are enclosed in single quotation marks.
C++ Declare String Variable
'C' also allows us to initialize a string variable without defining the size of the character array. It can be done in the following way,
The name of a string acts as a pointer because it is basically an array.
String Input: Read a String
When writing interactive programs which ask the user for input, C provides the scanf(), gets(), and fgets() functions to find a line of text entered from the user.
When we use scanf() to read, we use the '%s' format specifier without using the '&' to access the variable address because an array name acts as a pointer. For example:
How To Declare A String In Dev C Download
Output:
The problem with the scanf function is that it never reads an entire string. It will halt the reading process as soon as whitespace, form feed, vertical tab, newline or a carriage return occurs. Suppose we give input as 'Guru99 Tutorials' then the scanf function will never read an entire string as a whitespace character occurs between the two names. The scanf function will only read Guru99.
In Short, Your self merely incorporate in the direction of work the Addictive Lead to and on your own are geared up for the magnificent phase it deals. Vst crack waves 10 crack. Develop exclusive, abundant, and pristine seems. Waves 11 Full Bundle Free Download Full Version LatestThis software package can be applied for fixing music information as effectively as for burning substitute tunes upon CDs.
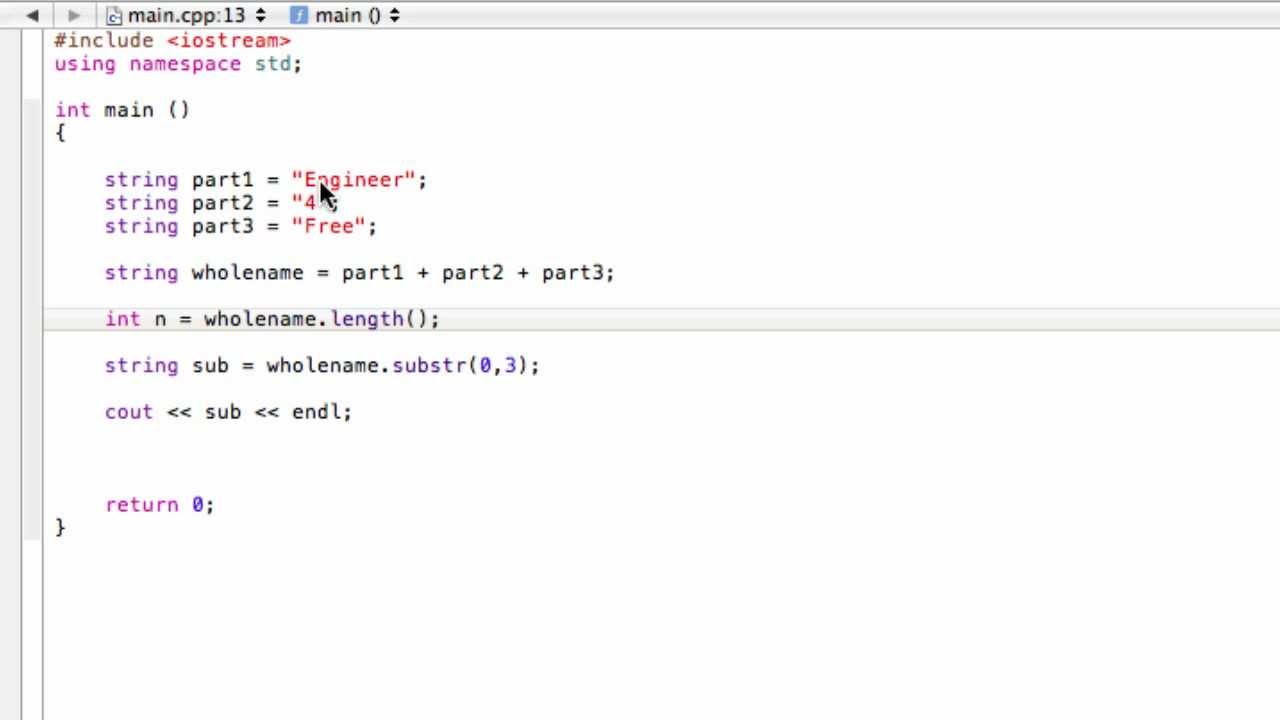
In order to read a string contains spaces, we use the gets() function. Gets ignores the whitespaces. It stops reading when a newline is reached (the Enter key is pressed).For example:
Output:
Another safer alternative to gets() is fgets() function which reads a specified number of characters. For example:
Output:
The fgets() arguments are :
- the string name,
- the number of characters to read,
- stdin means to read from the standard input which is the keyboard.
String Output: Print/Display a String
The standard printf function is used for printing or displaying a string on an output device. The format specifier used is %s
Example,
String output is done with the fputs() and printf() functions.
fputs() function
The fputs() needs the name of the string and a pointer to where you want to display the text. We use stdout which refers to the standard output in order to print to the screen.For example:
Output:
Get reviews, hours, directions, coupons and more for Precision Tune Auto Care at 2620 S Dairy Ashford Rd (Energy Corridor), Houston, TX 77082. Search for other Auto Repair & Service in Houston on The. Merry Christmas from Precision Tune 2 Houston TX now has two Precision Tune Auto Care Locations.Dairy Ashford area.Copperfield (newest location) Congratulations to Franchisee Sel Scherer and the. Precision tune auto care south dairy ashford road houston tx usa 77041. Precision Tune Auto Care of Houston, Texas provides fast and affordable auto repair and maintenance. Let our certified technicians keep your vehicle safe and reliable. 2620 South Dairy Ashford Road, Houston, TX.
puts function
The puts function prints the string on an output device and moves the cursor back to the first position. A puts function can be used in the following way,
The syntax of this function is comparatively simple than other functions.
The string library
The standard 'C' library provides various functions to manipulate the strings within a program. These functions are also called as string handlers. All these handlers are present inside <string.h> header file.Function | Purpose |
strlen() | This function is used for finding a length of a string. It returns how many characters are present in a string excluding the NULL character. |
strcat(str1, str2) | This function is used for combining two strings together to form a single string. It Appends or concatenates str2 to the end of str1 and returns a pointer to str1. |
strcmp(str1, str2) | This function is used to compare two strings with each other. It returns 0 if str1 is equal to str2, less than 0 if str1 < str2, and greater than 0 if str1 > str2. |
Lets consider the program below which demonstrates string library functions:
Output:
Other important library functions are:
- strncmp(str1, str2, n) :it returns 0 if the first n characters of str1 is equal to the first n characters of str2, less than 0 if str1 < str2, and greater than 0 if str1 > str2.
- strncpy(str1, str2, n) This function is used to copy a string from another string. Copies the first n characters of str2 to str1
- strchr(str1, c): it returns a pointer to the first occurrence of char c in str1, or NULL if character not found.
- strrchr(str1, c): it searches str1 in reverse and returns a pointer to the position of char c in str1, or NULL if character not found.
- strstr(str1, str2): it returns a pointer to the first occurrence of str2 in str1, or NULL if str2 not found.
- strncat(str1, str2, n) Appends (concatenates) first n characters of str2 to the end of str1 and returns a pointer to str1.
- strlwr() :to convert string to lower case
- strupr() :to convert string to upper case
- strrev() : to reverse string
Java Declare String Variable
Converting a String to a Number
In C programming, we can convert a string of numeric characters to a numeric value to prevent a run-time error. The stdio.h library contains the following functions for converting a string to a number:
String In Dev C++
- int atoi(str) Stands for ASCII to integer; it converts str to the equivalent int value. 0 is returned if the first character is not a number or no numbers are encountered.
- double atof(str) Stands for ASCII to float, it converts str to the equivalent double value. 0.0 is returned if the first character is not a number or no numbers are encountered.
- long int atol(str) Stands for ASCII to long int, Converts str to the equivalent long integer value. 0 is returned if the first character is not a number or no numbers are encountered.
How To Declare A String In Dev C File
The following program demonstrates atoi() function:
Output:
- A string pointer declaration such as char *string = 'language' is a constant and cannot be modified.
C++ Declare Array Of Strings
Summary
Declare A String Java
- A string is a sequence of characters stored in a character array.
- A string is a text enclosed in double quotation marks.
- A character such as 'd' is not a string and it is indicated by single quotation marks.
- 'C' provides standard library functions to manipulate strings in a program. String manipulators are stored in <string.h> header file.
- A string must be declared or initialized before using into a program.
- There are different input and output string functions, each one among them has its features.
- Don't forget to include the string library to work with its functions
- We can convert string to number through the atoi(), atof() and atol() which are very useful for coding and decoding processes.
- We can manipulate different strings by defining a string array.